Location
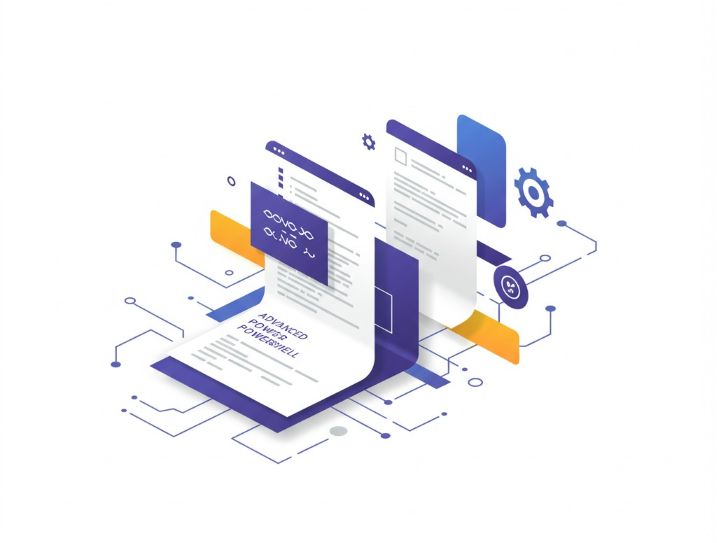
PowerShell is a powerful scripting language and automation framework widely used by IT professionals, developers, and system administrators. While beginners may focus on basic cmdlets and scripts, advanced PowerShell scripting opens up a realm of possibilities for tackling complex tasks and automating intricate workflows. This article explores expert-level tips and tricks to help you elevate your PowerShell scripting skills to the next level.
Understanding Advanced PowerShell Concepts
Harnessing the Power of Functions
Functions are at the heart of reusable, modular, and efficient PowerShell scripts. They allow you to break down complex scripts into smaller, manageable units. Here\’s an example of creating a function with parameters and a return value:
function Get-Square {
param (
[int]$Number
)
return $Number * $Number
}
# Example Usage
Get-Square -Number 4
Using Script and Module Scope
When working on large projects, understanding scope is critical. PowerShell has various scopes, such as local, script, and global. Properly managing variables and functions across these scopes ensures modularity and prevents conflicts.
- Script Scope: Variables declared in this scope are accessible throughout the script.
- Module Scope: Used within custom modules to manage access to variables and functions.
Creating and Using PowerShell Modules
Modules are essential for organizing and sharing scripts. They bundle functions, cmdlets, and resources into reusable packages. Here\’s how to create a module:
- Save your functions in a
.psm1
file. - Include a manifest file (
.psd1
) to define module metadata.
Example:
# MyModule.psm1
function Say-Hello {
param ([string]$Name)
Write-Output \"Hello, $Name!\"
}
# Manifest: MyModule.psd1
@{
ModuleVersion = \'1.0.0\'
FunctionsToExport = \'Say-Hello\'
}
Error Handling with Try-Catch-Finally
Advanced scripting requires robust error handling. The try-catch-finally
construct helps manage exceptions gracefully:
try {
Get-Item \'C:\\NonExistentFile.txt\'
} catch {
Write-Output \"Error: $($_.Exception.Message)\"
} finally {
Write-Output \"Execution completed.\"
}
Leveraging Advanced Features in PowerShell
Working with the Pipeline
PowerShell\’s pipeline enables seamless data processing. Advanced usage includes:
- Filtering: Use
Where-Object
for conditional processing. - Custom Objects: Create structured outputs for complex pipelines.
Example:
Get-Process | Where-Object { $_.CPU -gt 100 } | Select-Object Name, CPU
Utilizing Classes in PowerShell
PowerShell 5.0 introduced classes, enabling object-oriented programming (OOP). You can create classes with properties, methods, and constructors:
class Car {
[string]$Make
[string]$Model
Car ([string]$Make, [string]$Model) {
$this.Make = $Make
$this.Model = $Model
}
[string]Drive() {
return \"$($this.Make) $($this.Model) is driving!\"
}
}
$myCar = [Car]::new(\'Tesla\', \'Model S\')
$myCar.Drive()
Harnessing PowerShell Remoting
PowerShell remoting enables executing scripts on remote systems securely. Use Invoke-Command
to run commands on one or multiple remote machines:
Invoke-Command -ComputerName Server1, Server2 -ScriptBlock {
Get-Service -Name \'W32Time\'
}
Using Runspaces for Parallel Processing
Runspaces allow you to execute multiple tasks simultaneously, improving efficiency in large-scale automation:
$RunspacePool = [runspacefactory]::CreateRunspacePool(1, 5)
$RunspacePool.Open()
$Jobs = @()
1..10 | ForEach-Object {
$Job = [powershell]::Create().AddScript({ $_ * $_ })
$Job.RunspacePool = $RunspacePool
$Job.BeginInvoke()
$Jobs += $Job
}
$Jobs | ForEach-Object { $_.EndInvoke() }
$RunspacePool.Close()
Tips for Writing Cleaner, Efficient Scripts
Use Comments and Documentation
Well-commented scripts are easier to maintain and share. Use inline comments and PowerShell Help to document functions:
<#
.SYNOPSIS
Calculates the square of a number.
.DESCRIPTION
This function takes an integer input and returns its square.
.EXAMPLE
Get-Square -Number 5
#>
function Get-Square {
param ([int]$Number)
return $Number * $Number
}
Follow Naming Conventions
Adopt consistent naming conventions for variables, functions, and files:
- Functions: Verb-Noun (e.g.,
Get-Process
,Set-Configuration
) - Variables: Use meaningful names (e.g.,
$FilePath
,$UserList
)
Optimize Performance
- Avoid Unnecessary Loops: Use PowerShell cmdlets like
Where-Object
andForEach-Object
. - Use Native Cmdlets: Built-in cmdlets are optimized for performance.
- Filter Early: Fetch only required data to reduce memory overhead.
Security Best Practices
Secure Credentials
Avoid hardcoding credentials in scripts. Use Get-Credential
or secure strings:
$Credential = Get-Credential
Invoke-Command -ComputerName Server1 -Credential $Credential -ScriptBlock {
Get-Process
}
Code Signing
Sign your scripts with a trusted certificate to ensure authenticity:
Set-AuthenticodeSignature -FilePath .\\MyScript.ps1 -Certificate $Cert
Use Execution Policies
Set appropriate execution policies to control script execution:
Set-ExecutionPolicy RemoteSigned
Debugging and Troubleshooting
Using the Debugger
PowerShell includes a built-in debugger to analyze scripts line by line:
Set-PSBreakpoint -Script MyScript.ps1 -Line 10
Logging and Verbose Output
Implement logging to track script activity and errors:
Write-Verbose \"Processing file: $FilePath\" -Verbose
Profiling Scripts
Measure script performance using the Measure-Command
cmdlet:
Measure-Command {
Get-ChildItem -Path C:\\Windows -Recurse
}
Conclusion
Advanced PowerShell scripting unlocks powerful capabilities for IT professionals and developers. By leveraging functions, modules, error handling, and advanced features like remoting and parallel processing, you can build efficient, secure, and maintainable scripts. Follow best practices, optimize for performance, and continue exploring PowerShell\’s vast potential to stay ahead in your scripting journey.